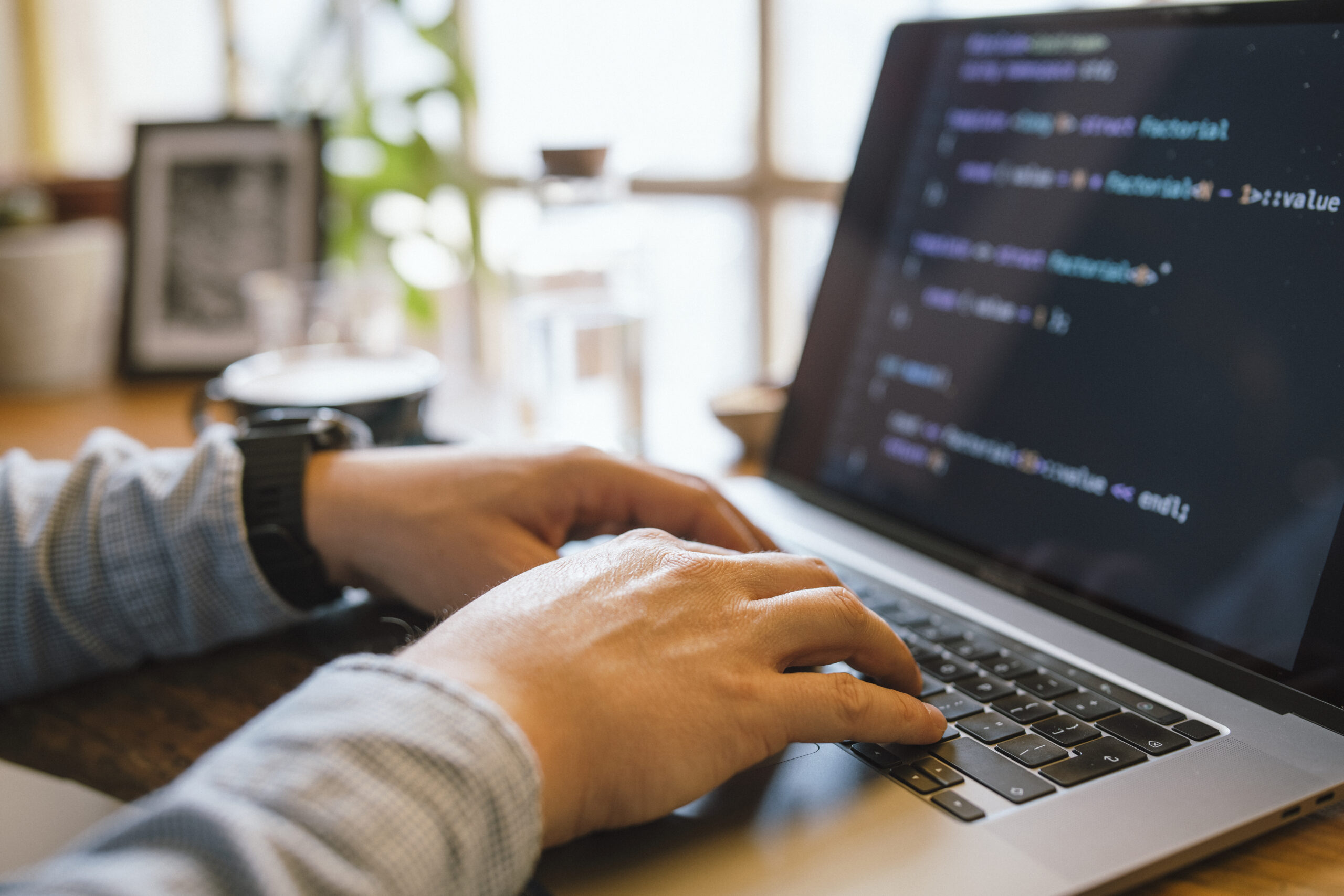
Debugging is The most necessary — yet usually neglected — competencies in a developer’s toolkit. It's not just about fixing broken code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve troubles efficiently. Whether or not you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of stress and substantially increase your productiveness. Listed below are numerous techniques that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of quickest means builders can elevate their debugging expertise is by mastering the resources they use daily. Whilst composing code is a single part of enhancement, figuring out the way to interact with it properly in the course of execution is Similarly significant. Modern day improvement environments occur Outfitted with strong debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Consider, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments allow you to established breakpoints, inspect the value of variables at runtime, step as a result of code line by line, and in many cases modify code within the fly. When used properly, they let you observe exactly how your code behaves through execution, which can be a must have for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-stop developers. They enable you to inspect the DOM, watch network requests, see serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can transform discouraging UI concerns into workable jobs.
For backend or method-stage developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Management above functioning processes and memory administration. Understanding these instruments may have a steeper Finding out curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, grow to be at ease with version Regulate units like Git to be aware of code background, obtain the exact moment bugs have been launched, and isolate problematic variations.
Ultimately, mastering your resources usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your enhancement atmosphere to ensure when difficulties crop up, you’re not lost at nighttime. The greater you recognize your instruments, the greater time you'll be able to shell out solving the particular challenge in lieu of fumbling through the process.
Reproduce the trouble
Just about the most essential — and sometimes forgotten — techniques in productive debugging is reproducing the situation. In advance of leaping in to the code or producing guesses, developers want to create a dependable natural environment or circumstance in which the bug reliably appears. Without having reproducibility, fixing a bug results in being a video game of possibility, typically leading to squandered time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you have got, the much easier it turns into to isolate the precise problems under which the bug takes place.
As soon as you’ve collected enough facts, attempt to recreate the problem in your neighborhood atmosphere. This may signify inputting the identical details, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, think about producing automated assessments that replicate the sting circumstances or point out transitions involved. These assessments not just enable expose the issue and also prevent regressions Sooner or later.
In some cases, the issue may be setting-unique — it might transpire only on certain running devices, browsers, or beneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But once you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, you can use your debugging equipment additional proficiently, exam potential fixes safely, and communicate much more Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers must discover to treat error messages as immediate communications with the technique. They generally let you know just what happened, where by it happened, and in some cases even why it took place — if you know how to interpret them.
Start by examining the concept cautiously As well as in full. Quite a few developers, especially when less than time strain, glance at the 1st line and right away commence making assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste error messages into search engines like google — examine and realize them initial.
Crack the error down into sections. Is it a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some faults are obscure or generic, As well as in those circumstances, it’s very important to examine the context through which the mistake happened. Check out related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized troubles and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful instruments in a very developer’s debugging toolkit. When utilized efficiently, it offers genuine-time insights into how an software behaves, assisting you fully grasp what’s going on under the hood with no need to pause execution or phase throughout the code line by line.
A superb logging tactic commences with recognizing what to log and at what amount. Common logging levels consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of progress, Data for basic occasions (like effective start-ups), Alert for likely troubles that don’t break the appliance, ERROR for genuine challenges, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant facts. A lot of logging can obscure essential messages and decelerate your technique. Give attention to key situations, condition changes, input/output values, and significant selection details with your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in output environments exactly where stepping by code isn’t feasible.
Additionally, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, you can reduce the time it will require to identify problems, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of one's code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To successfully discover and repair bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking allows stop working intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indications of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as you'll be able to without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Next, type hypotheses. Inquire your self: What could possibly be leading to this behavior? Have any changes recently been built into the codebase? Has this challenge happened in advance of beneath equivalent situations? The goal should be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Seek to recreate the situation in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest specifics. Bugs often cover inside the the very least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race condition. Be extensive and patient, resisting the urge to patch The problem without thoroughly knowing it. Temporary fixes may well hide the true problem, just for it to resurface later.
And lastly, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and support others fully grasp your reasoning.
By pondering similar to a detective, developers can sharpen their analytical skills, tactic issues methodically, and come to be more effective at uncovering concealed problems in intricate methods.
Publish Checks
Creating exams is among the most effective approaches to transform your debugging competencies and Total enhancement efficiency. Tests not just support capture bugs early and also function a security Web that offers you self-confidence when making modifications in your codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Start with unit checks, which deal with individual capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Performing as predicted. Every time a take a look at fails, you quickly know in which to search, considerably decreasing some time used debugging. Device exams are Particularly beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support be sure that a variety of elements of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that occur in advanced techniques with multiple parts or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically regarding your code. To check a aspect appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails consistently, you'll be able to deal with fixing the bug and look at your exam pass when The problem is fixed. This strategy makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a annoying guessing video game right into a structured and predictable procedure—aiding you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Remedy immediately after solution. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, cut down frustration, and often see The difficulty from the new standpoint.
If you're much too close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours before. In this point out, your Mind gets considerably less successful at challenge-fixing. A short walk, a espresso split, as well as switching to a distinct job for 10–quarter-hour can refresh your emphasis. A lot of developers report finding the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a display, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or Gustavo Woltmann coding do anything unrelated to code. It may come to feel counterintuitive, Specifically less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each and every bug you face is a lot more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful when you go to the trouble to reflect and analyze what went Improper.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log in which you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively stay away from.
In group environments, sharing what you've acquired from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. After all, many of the very best builders aren't those who write best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to be better at Everything you do.